Mastering Data Fetching in React with SWR: The Comprehensive Guide
Written on
Introduction to SWR
Are you looking for a more effective way to retrieve data in your React applications? In this guide, we will delve into SWR, a powerful hook that transforms data fetching, providing developers with enhanced speed and flexibility. SWR introduces a distinctive method for data fetching and synchronization, paving the way for the creation of swift, reliable, and real-time applications.
What You Will Learn
- Utilizing SWR for data fetching in React applications
- Configuring SWR options
- Managing errors with SWR
By the end of this guide, you will be equipped with the skills to develop more resilient and interactive applications, unlocking a new level of functionality in your developer toolkit.
Benefits of SWR
SWR, which stands for Stale-While-Revalidate, is a strategy derived from HTTP RFC 5861. This approach allows your application to render stale data while asynchronously fetching fresh data in the background, ultimately providing users with updated information.
Advantages of SWR
Immediate Rendering and Real-time Updates
SWR enables your application to display cached (stale) data immediately, followed by background updates with fresh data. This instant display greatly enhances the user experience.
Automatic Retries and Polling
With built-in automatic retries, SWR will persistently attempt to fetch data in case of network failures. Additionally, you can set SWR to periodically poll your server for real-time updates.
Prerequisites for SWR
Before using SWR, ensure that Node.js and npm are installed on your system. You can initialize a new React project using tools like create-react-app, Next.js, or set it up manually.
How to Fetch Data with SWR
To incorporate SWR into your React project, install it using the following commands:
npm install swr
or
yarn add swr
Next, employ the useSWR hook in your component to retrieve data:
import useSWR from 'swr';
function Profile() {
const { data, error, isLoading } = useSWR('/api/user/123', fetcher);
if (error) return <div>Failed to load</div>;
if (isLoading) return <div>Loading...</div>;
return <div>Hello {data.name}!</div>;
}
In this example, useSWR takes two arguments: the URL to fetch and the fetcher function. The hook returns an object with the fetched data and any potential errors.
SWR Configuration Options
SWR offers various configuration options to customize your data fetching behavior. You can provide these settings as the third argument to the useSWR hook or set them globally for all calls.
Common Configuration Options:
- refreshInterval: Defines the polling interval (in milliseconds).
- revalidateOnFocus: Specifies whether SWR should revalidate data when the window regains focus.
- errorRetryCount: Sets the maximum number of retries upon encountering an error.
Error Handling with SWR
SWR has robust error-handling capabilities. If an error occurs during data fetching, the useSWR hook will provide an error object for you to manage.
import useSWR from 'swr';
function Profile() {
const { data, error, isLoading } = useSWR('/api/user/123', fetcher);
if (error) return <div>Failed to load: {error.message}</div>;
if (isLoading) return <div>Loading...</div>;
return <div>Hello {data.name}!</div>;
}
In this snippet, we check for errors using if (error) and display the error message accordingly. While loading, we show a loading indicator, and once data retrieval is complete, we display the fetched information.
Conclusion
Effective data fetching is crucial for delivering a seamless user experience. The SWR hook offers a revolutionary approach to data retrieval, equipping developers with a powerful, versatile tool for fetching, synchronizing, and updating data in real time. This advancement enhances the overall developer experience, resulting in more elegant applications.
Thank you for exploring data fetching with SWR. I hope this guide has been informative and beneficial for your web development journey. Should you have any startup ideas, feel free to reach out via futuromy.com—I can help validate and build your startup concept!
For additional insights, you can check out similar articles. If you have any questions or feedback, don't hesitate to drop me a message!
Follow me on Twitter for more updates!
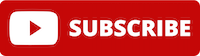
Chapter 2: Video Resources
In this chapter, we’ll explore some valuable video resources to deepen your understanding of SWR.
Discover the fundamentals of SWR and how it can enhance your data-fetching strategy in React applications.
Learn the best practices for fetching data from APIs in React using SWR, including preload and optimistic UI techniques.
Happy Coding!
Melih