Understanding the Oversight in Java Serialization: A Deep Dive
Written on
Chapter 1: The Significance of Serialization in Java
Serialization in Java enables the transformation of an object into a byte stream, facilitating storage or transmission over a network. This process is crucial in microservices architectures, where exchanging objects between services frequently necessitates serialization and deserialization.
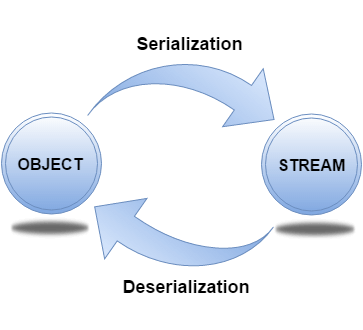
Nevertheless, developers frequently overlook a critical aspect of implementing the Java Serializable interface—the serialVersionUID field. This field acts as a version control mechanism, ensuring that both serialized and deserialized objects are compatible.
Section 1.1: The Absence of serialVersionUID
Consider the following example without the serialVersionUID defined. Suppose we create a Person class that implements the Serializable interface, but we neglect to declare the serialVersionUID field:
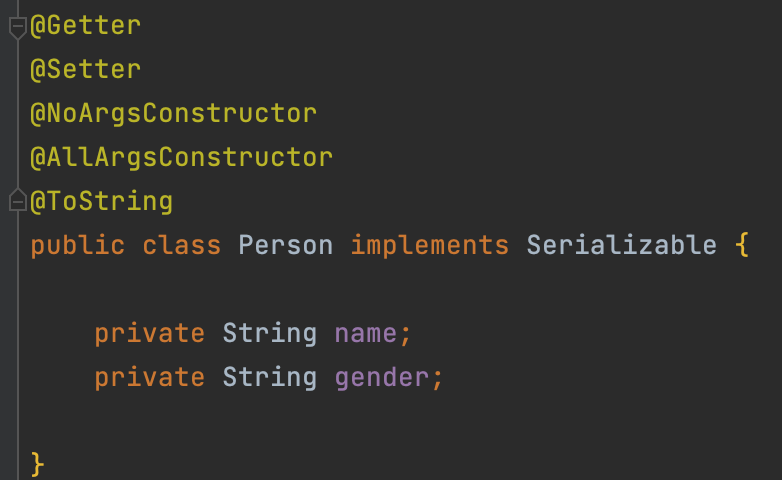
Let's implement a method to serialize a Person object:
public void serialize() {
Person person = new Person();
person.setName("DN Tech");
person.setGender("F");
try {
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("person.ser"));
oos.writeObject(person);
} catch (IOException e) {
throw new RuntimeException(e);}
}
Upon executing this method, the “person.ser” file will contain a series of unreadable characters, potentially leading to encoding issues.

Section 1.2: Attempting Deserialization
Do not be alarmed; let's proceed to deserialize the same file:
public void deserialize() {
try {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("person.ser"));
Person person = (Person) ois.readObject();
System.out.println(person);
} catch (Exception e) {
throw new RuntimeException(e);}
}
After execution, we find that the previously created Person object is successfully printed:
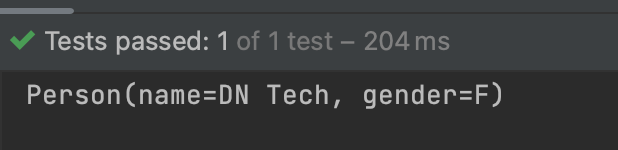
Now, suppose we decide to add an additional field, "mobile", to the Person class.
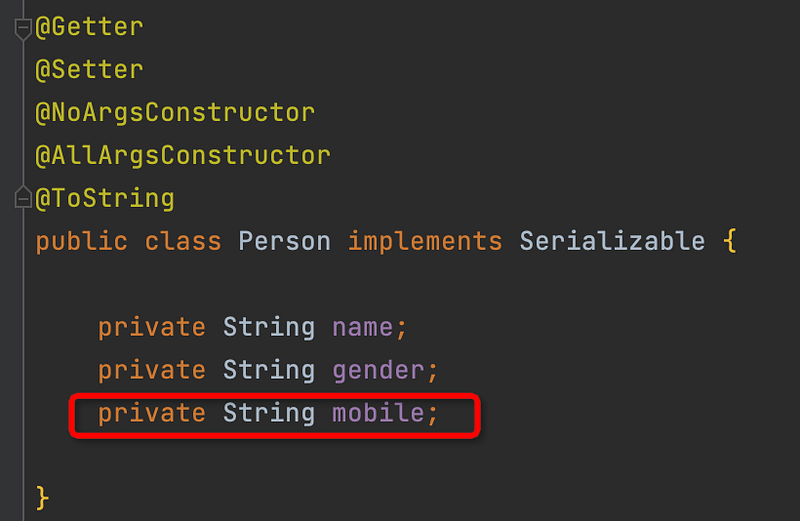
Let’s run the deserialize method once more.

Section 1.3: The InvalidClassException
What just happened? We received an InvalidClassException, indicating a mismatch in serialVersionUID. The reason for this is that modifying the Person class prompts the JVM to generate a new serialVersionUID, as illustrated with the local class identifier. The previously serialized file retains the original serialVersionUID. Consequently, when the two versions do not align, the JVM cannot perform deserialization.
Imagine the complications this could introduce in our software systems, where older objects become irretrievable after modifications.
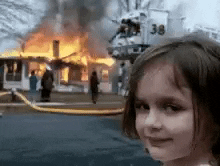
Chapter 2: Emphasizing serialVersionUID
Fortunately, both Eclipse and IntelliJ IDEA provide built-in methods to generate the serialVersionUID for us.
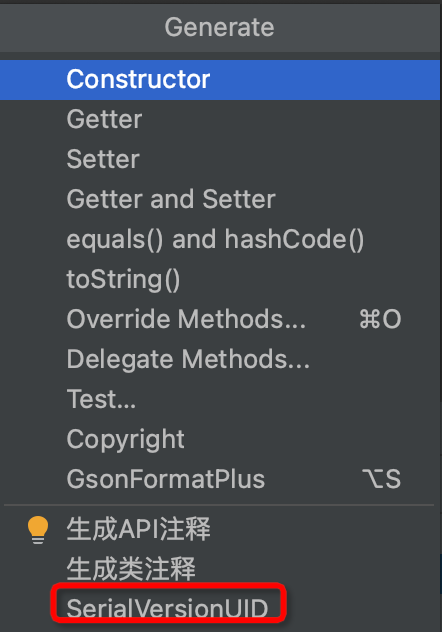
Now that we have a defined serialVersionUID for the class, we can proceed without the concern of version conflicts.
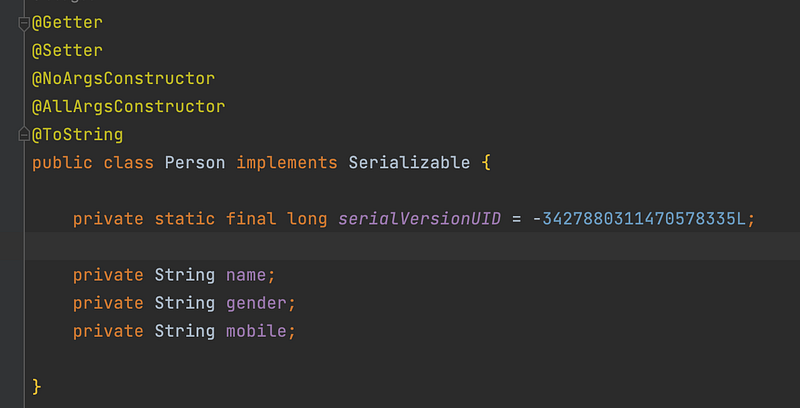
Let’s attempt to serialize the object again:
public void serialize() {
Person person = new Person();
person.setName("DN Tech");
person.setGender("F");
person.setMobile("00000000");
try {
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("person.ser"));
oos.writeObject(person);
} catch (IOException e) {
throw new RuntimeException(e);}
}
Next, we will add an "address" field to the Person object and check if it can be successfully deserialized.
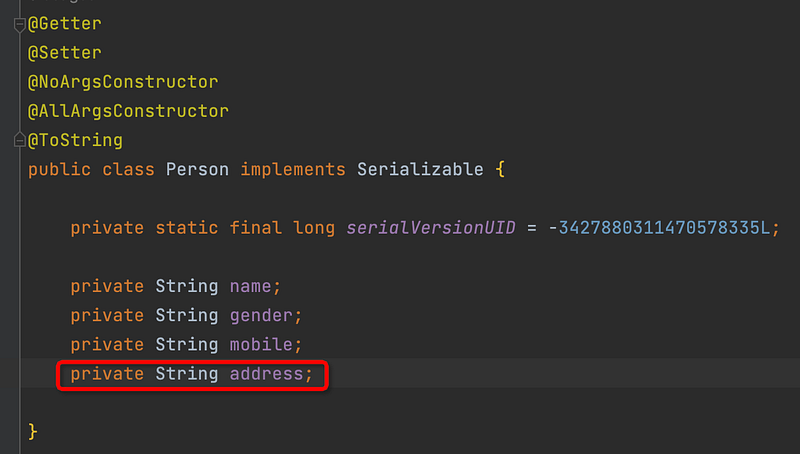
Result:

Indeed! Since the previously serialized Person did not possess an address value, deserialization correctly assigns it a null value, ensuring compatibility with older objects.
I trust this article has been insightful. As a backend software engineer, I invite those interested in technology to follow my channel for ongoing updates and inspirations from my professional journey.
Get Connected:
My LinkedIn
For further reading, check out:
My Favorite 5 IntelliJ Plugins That Can Boost Your Productivity
IntelliJ has become the most popular IDE for Java development in 2022.
blog.devgenius.io
10 Best Software Engineering Practices for Java
- Write meaningful Java-docs/comments
10 Java Shortcuts in IntelliJ IDEA
My previous article on my favorite IntelliJ plugins received fantastic feedback.
blog.devgenius.io
The first video titled "Why We Hate Java Serialization And What We're Doing About It" by Brian Goetz & Stuart Marks discusses common issues with Java serialization and potential solutions.
The second video, "Java Serialization was a Horrible Mistake," explores the pitfalls of Java serialization and highlights the need for better practices in the field.